面向切面编程AOP
1.Spring的AOP简介
1.1什么是AOP
AOP为Aspect Oriented Programming的缩写,意思是面向切面编程,是通过预编译和运行期动态代理实现程序功能维护的一种技术
AOP是OOP(面向对象)的延续,利用AOP可以对业务逻辑的各部分进行隔离,从而降低业务逻辑之间的耦合度,提高程序的可重用性,同时提高开发效率
1.2.AOP的作用及优势
作用:在程序运行期间,在不修改源码的情况下对方法进行功能增强
优势:减少重复代码,提高开发效率,便于维护
1.3AOP底层实现
AOP底层是通过Spring提供的动态代理技术实现的,Spring通过动态代理技术动态动态的生成代理对象,代理对象方法执行时进行增强功能介入
1.4 AOP的动态代理技术
JDK代理:基于接口的动态代理技术
cglib代理:基于父类的动态代理技术
1.5JDK的动态代理
(1)目标接口
public interface TargetInterface {public void method();
}
(2)目标类
public class Target implements TargetInterface {@Overridepublic void method() {System.out.println("Target running....");}
}
(3)动态代理代码
Target target = new Target(); //创建目标对象//创建代理对象TargetInterface proxy = (TargetInterface) Proxy.newProxyInstance(target.getClass().getClassLoader(),target.getClass().getInterfaces(),new InvocationHandler() {@Overridepublic Object invoke(Object proxy, Method method, Object[] args) throws Throwable {System.out.println("前置增强代码...");Object invoke = method.invoke(target, args);System.out.println("后置增强代码...");return invoke;}}
);
(4)调用代理对象的方法测试
proxy.method();
1.6 cglib
(1)目标类
public class Target {public void method() {System.out.println("Target running....");}
}
(2) 动态代理代码
Target target = new Target(); //创建目标对象Enhancer enhancer = new Enhancer(); //创建增强器enhancer.setSuperclass(Target.class); //设置父类enhancer.setCallback(new MethodInterceptor() { //设置回调@Overridepublic Object intercept(Object o, Method method, Object[] objects, MethodProxy methodProxy) throws Throwable {System.out.println("前置代码增强....");Object invoke = method.invoke(target, objects);System.out.println("后置代码增强....");return invoke;}});
Target proxy = (Target) enhancer.create(); //创建代理对象
(3) 调用代理对象的方法测试
proxy.method();
1.7 AOP相关概念
Spring的AOP实现底层就是对上面的动态代理的代码进行了封装,封装后我们只需要对需要关注的部分进行代码编写,并通过配置的方式完成指定的方法增强
Target(目标对象):代理的目标对象
Proxy(代理):一个类被AOP织入增强后,就产生一个结果代理类
Joinpoint(连接点):所谓连接点是指那些 被拦截到的点,在Spring中这些点指的是方法
Pointcut(切入点):所谓切入点是指我们要对那些Joinpoint进行拦截定义
Advice(通知/增强):所谓通知是指拦截到Joinpoint之后要做的事情就是通知
Aspect(切面):是切入点和通知的结合
Weaving(织入):是指把增强应用到目标对象来创建新的代理对象的过程
2.基于XML的AOP开发
2.1 快速入门
(1)导入AOP相关坐标
<!--导入spring的context坐标,context依赖aop-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.5.RELEASE</version>
</dependency>
<!-- aspectj的织入 -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.13</version>
</dependency
(2)创建目标接口和目标类
public interface TargetInterface {public void method();
}
public class Target implements TargetInterface {@Overridepublic void method() {System.out.println("Target running....");}
}
(3) 创建切面类(内部有增强方法)
public class MyAspect {//前置增强方法public void before(){System.out.println("前置代码增强.....");}
}
(4)将目标类和切面类的对象创建权交给Spring
<!--配置目标类-->
<bean id="target" class="com.lin.aop.Target"></bean>
<!--配置切面类-->
<bean id="myAspect" class="com.lin.aop.MyAspect"></bean>
(5)在applicationContext.xml中导入aop的命名空间
<beans xmlns="http://www.springframework.org/schema/beans"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xmlns:context="http://www.springframework.org/schema/context"xmlns:aop="http://www.springframework.org/schema/aop"xsi:schemaLocation="http://www.springframework.org/schema/contexthttp://www.springframework.org/schema/context/spring-context.xsdhttp://www.springframework.org/schema/aophttp://www.springframework.org/schema/aop/spring-aop.xsdhttp://www.springframework.org/schema/beanshttp://www.springframework.org/schema/beans/spring-beans.xsd">
在applicationContext.xml中配置织入关系
配置切点表达式和前置增强的织入关系
<aop:config><!--引用myAspect的Bean为切面对象--><aop:aspect ref="myAspect"><!--配置Target的method方法执行时要进行myAspect的before方法前置增强--><aop:before method="before" pointcut="execution(public void com.lin.aop.Target.method())"></aop:before></aop:aspect>
</aop:config>
(6)测试代码
@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration("classpath:applicationContext.xml")public class AopTest {@Autowiredprivate TargetInterface target;@Testpublic void test1(){target.method();}
}
2.2 xml配置AOP详解
1.切点表达式的写法
execution([修饰符] 返回值类型 包名.类名.方法名(参数))
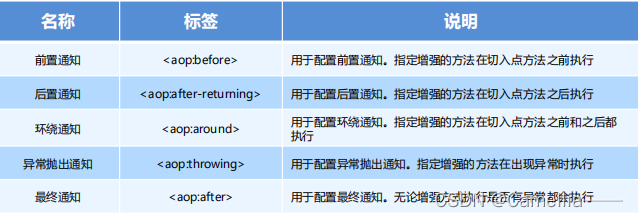
3.切点表达式的抽取
当多个增强切点表达式相同时,可以将切点表达式进行抽取,在增强中使用pointcut属性来抽取切点表达式
<aop:config><!--引用myAspect的Bean为切面对象--><aop:aspect ref="myAspect"><aop:pointcut id="myPointcut" expression="execution(* com.lin.aop.*.*(..))"/><aop:before method="before" pointcut-ref="myPointcut"></aop:before></aop:aspect>
</aop:config
2.3要点
aop织入的配置
<aop:config><aop:aspect ref=“切面类”><aop:before method=“通知方法名称” pointcut=“切点表达式"></aop:before></aop:aspect>
</aop:config>
3.基于AOP的注解开发
3.1快速入门
(1)创建接口和目标类
public interface TargetInterface {public void method();
}
public class Target implements TargetInterface {@Overridepublic void method() {System.out.println("Target running....");}
}
(2)创建切面类
public class MyAspect {//前置增强方法public void before(){System.out.println("前置代码增强.....");}
}
(3)将目标类和切面类的对象创建权交给spring
@Component("target")
public class Target implements TargetInterface {@Overridepublic void method() {System.out.println("Target running....");}
}
@Component("myAspect")
public class MyAspect {public void before(){System.out.println("前置代码增强.....");}
}
(4)在切面类中使用注解配置织入关系
@Component("myAspect")
@Aspect
public class MyAspect {
@Before("execution(* com.itheima.aop.*.*(..))")
public void before(){System.out.println("前置代码增强.....");}
}
(5)在配置文件中开启组件扫描和AOP的自动代理
<!--组件扫描-->
<context:component-scan base-package="com.itheima.aop"/>
<!--aop的自动代理-->
<aop:aspectj-autoproxy></aop:aspectj-autoproxy>
(6)测试代码
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:applicationContext.xml")
public class AopTest {@Autowiredprivate TargetInterface target;@Testpublic void test1(){target.method();}
}
3.2注解配置AOP详解
@@Component("myAspect")
@Aspect
public class MyAspect {@Before("MyAspect.myPoint()")public void before(){System.out.println("前置代码增强.....");}
@Pointcut("execution(* com.itheima.aop.*.*(..))")public void myPoint(){}
}